Getting Started with TypeScript and React: A Beginner’s Guide
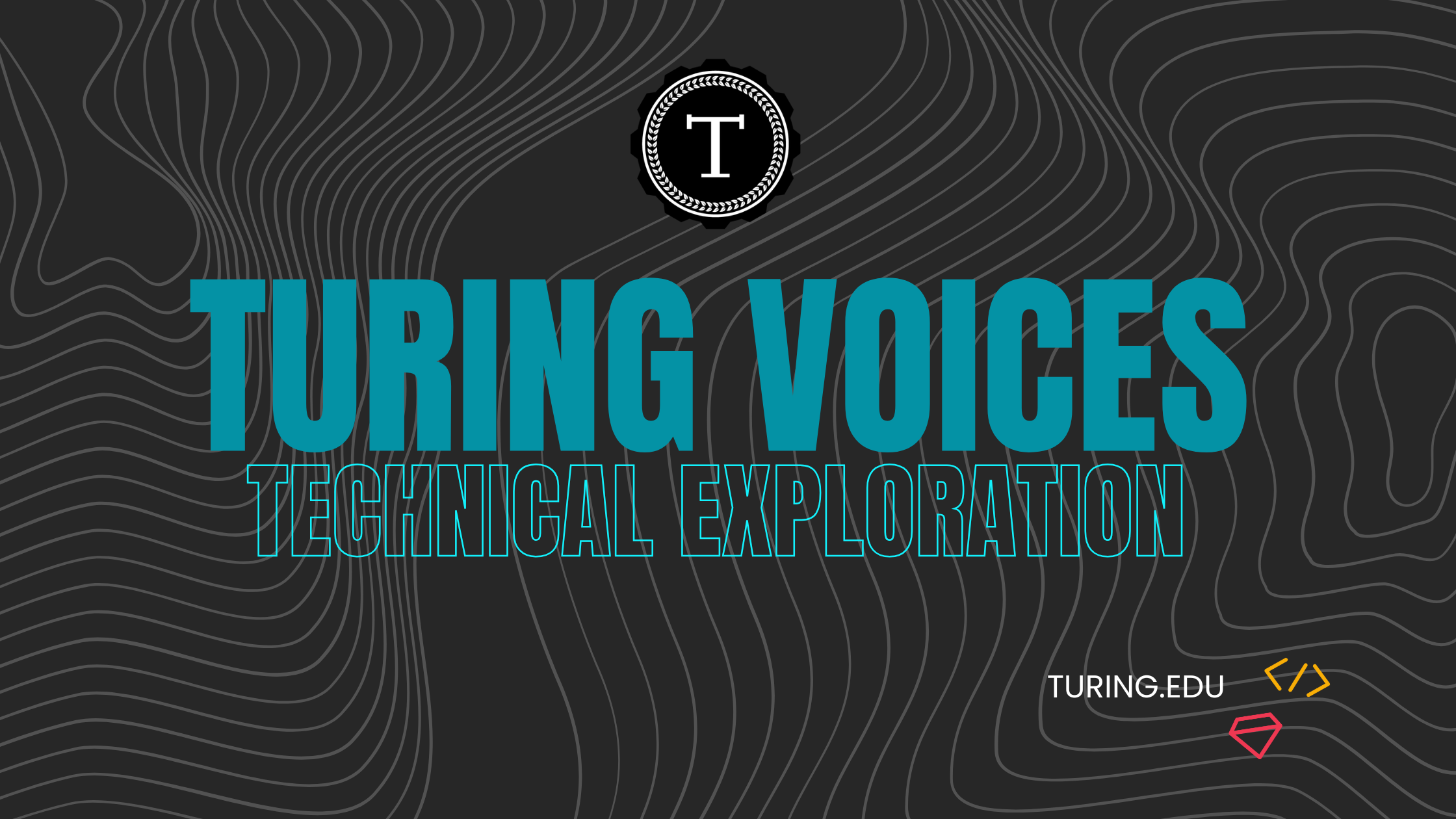
As React applications grow in complexity, managing data types and catching errors before runtime becomes increasingly important. This is where TypeScript comes into play. In this beginner-friendly guide, you’ll learn the essentials of TypeScript, how it integrates with React, and why it’s a game-changer for building scalable, error-free applications.
What is TypeScript?
TypeScript (TS) is an open-source superset of JavaScript (JS) that adds static typing and other features to make your code more robust and maintainable. Since TypeScript compiles to plain JavaScript, it works seamlessly with React, Node.js, and most modern web development tools.
Key Benefits of TypeScript
- Static Typing: Detects type-related errors during development, reducing runtime bugs.
- Improved IDE Support: Get better autocompletion, inline documentation, and error checking.
- Enhanced Readability: Clear type definitions make code easier to understand and maintain.
- Fewer Runtime Errors: Catch bugs early through compile-time checks.
⚙️ TypeScript vs. JavaScript: Key Differences
One of the biggest advantages of TypeScript is static typing, which prevents accidental type mismatches. Here’s a quick comparison:
JavaScript (Dynamic Typing)
let user = "Alex"; // A string
user = 10; // Now it's a number (valid in JS)
In JavaScript, variables can change types dynamically, which can lead to unexpected bugs.
TypeScript (Static Typing)
let user: string = "Alex";
user = 10; // ❌ Error: Type 'number' is not assignable to type 'string'
In TypeScript, once you declare a variable with a specific type, it must always hold that type.
💡 Why Use TypeScript with React?
When building React apps, using TypeScript offers several advantages:
- Type Safety:
Define strict types for your props, state, and components to prevent runtime errors. - Better Developer Experience:
IDE features like autocomplete and error highlighting make coding faster and more accurate. - Simplified Refactoring:
TypeScript makes refactoring easier by helping you identify and update all relevant types, reducing the risk of breaking your code. - Code Reliability:
TypeScript helps ensure that your app behaves as expected by catching more bugs during development.
TypeScript Workshop: Exploring TS Without React
Before diving into React with TypeScript, it’s helpful to practice TS syntax independently. This allows you to focus on TypeScript-specific concepts without the complexity of React.
🛠️ Explore with Repl.it
To get hands-on experience, check out this interactive Repl.it sandbox:
👉 TypeScript Intro on Repl.it
In this workshop, you’ll:
- Practice declaring variables with explicit types.
- Learn how TypeScript handles function parameters and return types.
- Explore type inference and how TypeScript automatically assigns types based on initial values.
Building a TypeScript React App
Once you’re comfortable with TypeScript fundamentals, it’s time to integrate it into a React app.
🛠️ Setting Up a New TypeScript React Project
To create a new React app with TypeScript from scratch, use the following command:
npx create-react-app my-app --template typescript
If you’re adding TypeScript to an existing React project, install the necessary dependencies:
npm install --save typescript @types/node @types/react @types/react-dom
TypeScript with React: Trivia App Project
To see TypeScript in action with React, try this Trivia App project, which uses the Open Trivia Database as its dataset. The app has some functionality built in—you’ll expand it and explore how TypeScript handles React components, props, and state.
🛠️ Explore the Trivia App Repo
Clone the project and run it locally:
👉 Trivia Repo on GitHub
What You’ll Learn
- Typing Props and State: Define strict types for props and component state.
- Using Interfaces: Leverage interfaces for better readability and reusability.
- Error Prevention: Catch potential issues before runtime with TypeScript’s static typing.
TypeScript in a React Component
Let’s see how TypeScript enhances a simple React component.
Typing Props
In JavaScript, you define props without specifying their type:
const Greeting = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
In TypeScript, you define the prop types using an interface:
interface GreetingProps {
name: string;
}
This ensures that name
must be a string. If you pass a number, you’ll get a compile-time error.
Common TypeScript React Patterns
Typing State and Hooks
When using useState
, you can specify the type of the state variable:
const [count, setCount] = useState<number>(0);
If you try to set count
to a string, TypeScript will catch the error:
setCount("five");
// ❌ Error: Type 'string' is not assignable to type 'number'
Defining Object Shapes
You can create custom types or interfaces to define object structures, improving code readability and consistency:
interface User {
id: number;
name: string;
isAdmin: boolean;
}
const user: User = {
id: 1,
name: "Alice",
isAdmin: true
};
Next Steps for Learning TypeScript
Once you’ve mastered the basics, take your TypeScript skills further by:
- Converting an Existing React Project to TypeScript:
Practice by gradually introducing TypeScript into one of your existing React projects. - Exploring Open-Source TypeScript Projects:
See how the pros use TypeScript in large-scale apps by contributing to open-source projects. - Learning TypeScript Generics:
Generics make your code more flexible and reusable by defining functions and components that work with multiple types. - Practicing TypeScript Interview Questions:
- What is the difference between
interface
andtype
in TypeScript? - How do you create and use custom types?
- Explain how TypeScript handles union and intersection types.
- What is the difference between
🌟 Top TypeScript Tools and Resources
Boost your TypeScript skills with these useful resources:
- Official TypeScript Docs – Comprehensive documentation and tutorials.
- React + TypeScript Cheatsheet – Common patterns and tips.
- TypeScript Playground – Test and experiment with TypeScript code online.
- YouTube Intro to TypeScript – Visual learning resources.
Turing School: Intro to Typescript with React
Final Thoughts
Learning TypeScript with React may feel overwhelming at first, but it offers long-term benefits by making your code safer, more readable, and easier to maintain. By gradually introducing TypeScript into your React projects, you’ll build stronger coding habits and become a more versatile developer.
Ready to take your TypeScript skills to the next level? Try building your own small project or convert an existing React app to TypeScript—it’s the best way to reinforce what you’ve learned! 🌟
This blog post was written by Danny Ramos
Be sure to follow us on Instagram, X, and LinkedIn - @Turing_School